Connect to data.world datasets using the JDBC driver dw-jdbc
dw-jdbc is a JDBC driver for connecting to datasets hosted on data.world. It can be used to provide read-only access to any dataset provided by data.world from any JVM language. dw-jdbc supports query access both in SQL (data.world's dialect - see details in our data.world SQL tutorial) and in SPARQL 1.1, the native query language for semantic web data sources.
JDBC URLs
JDBC connects to data source based on a provided JDBC url. data.world JDBC urls have the form
jdbc:data:world:[language]:[user id]:[dataset id]
where [language]
is either "sql" or "sparql",[user id]
is the data.world id of the dataset owner, and [dataset id]
is the data.world identifier for the dataset.
Sample code (Java 8)
final String QUERY = "select * from HallOfFame where playerID = ? order by yearid, playerID limit 10" final String URL = "jdbc:data:world:sql:dave:lahman-sabremetrics-dataset"; try (final Connection connection = // get a connection to the database, which will automatically be closed when done DriverManager.getConnection(URL, "<your user name>", "<your API token>"); final PreparedStatement statement = // get a connection to the database, which will automatically be closed when done connection.prepareStatement(QUERY)) { statement.setString(1, "alexape01"); //bind a query parameter try (final ResultSet resultSet = statement.executeQuery()) { //execute the query ResultSetMetaData rsmd = resultSet.getMetaData(); //print out the column headers int columnsNumber = rsmd.getColumnCount(); for (int i = 1; i <= columnsNumber; i++) { if (i > 1) System.out.print(", "); System.out.print(rsmd.getColumnName(i)); } System.out.println(""); while (resultSet.next()) { //loop through the query results for (int i = 1; i <= columnsNumber; i++) { //print out the column headers if (i > 1) System.out.print(", "); String columnValue = resultSet.getString(i); System.out.print(columnValue); } System.out.println(""); } } }
Building dw-jdbc
dw-jdbc can be built from the command-line using mvn clean install.
Finding your API tokens for data.world
When you need an API token for an integration, you can get it from your profile settings. Click on your user icon and choose Settings:
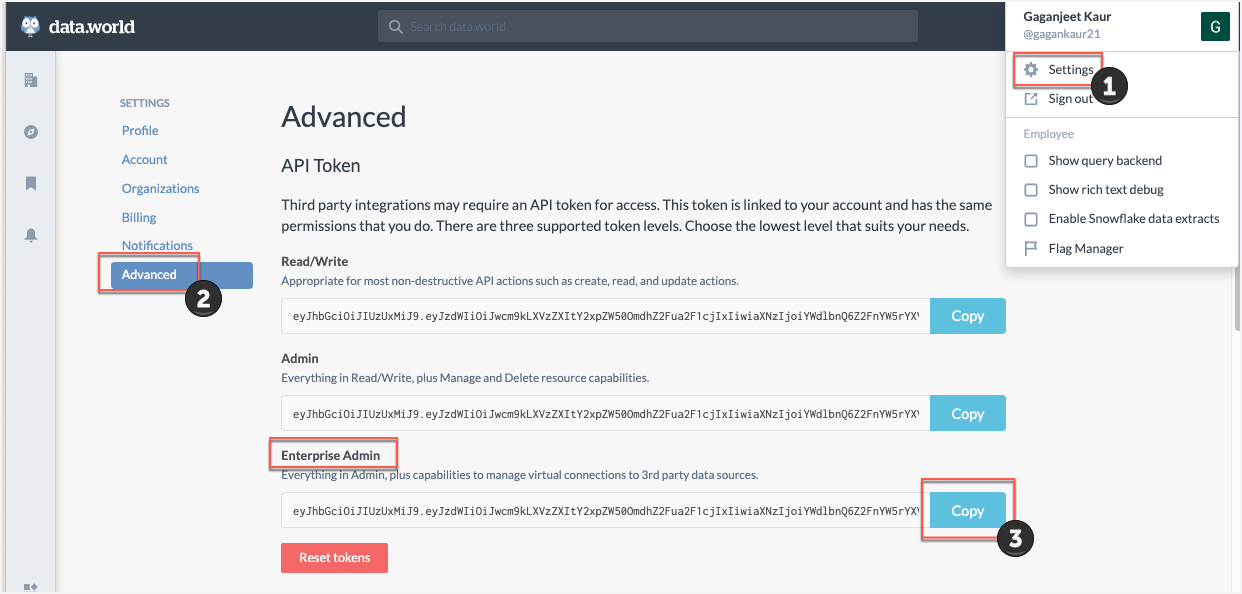
Both Read/Write and Admin tokens are provided. For the metadata catalog collector you can use the Read/Write token for the metadata catalog collector if you have write permissions to your organization's ddw-catalogs dataset.
More resources
More resources for using Java/JVM/JDBC-enabled tools: